Are you looking to supercharge your data analysis workflows with LangChain and CSV files? Read on to learn how to leverage CSVChain and LangChain for extracting insights from your comma-separated value data.
- For users who want to run a RAG system with no coding experience, you can try out Anakin AI, where you can create awesome AI Apps with a No Code Builder!
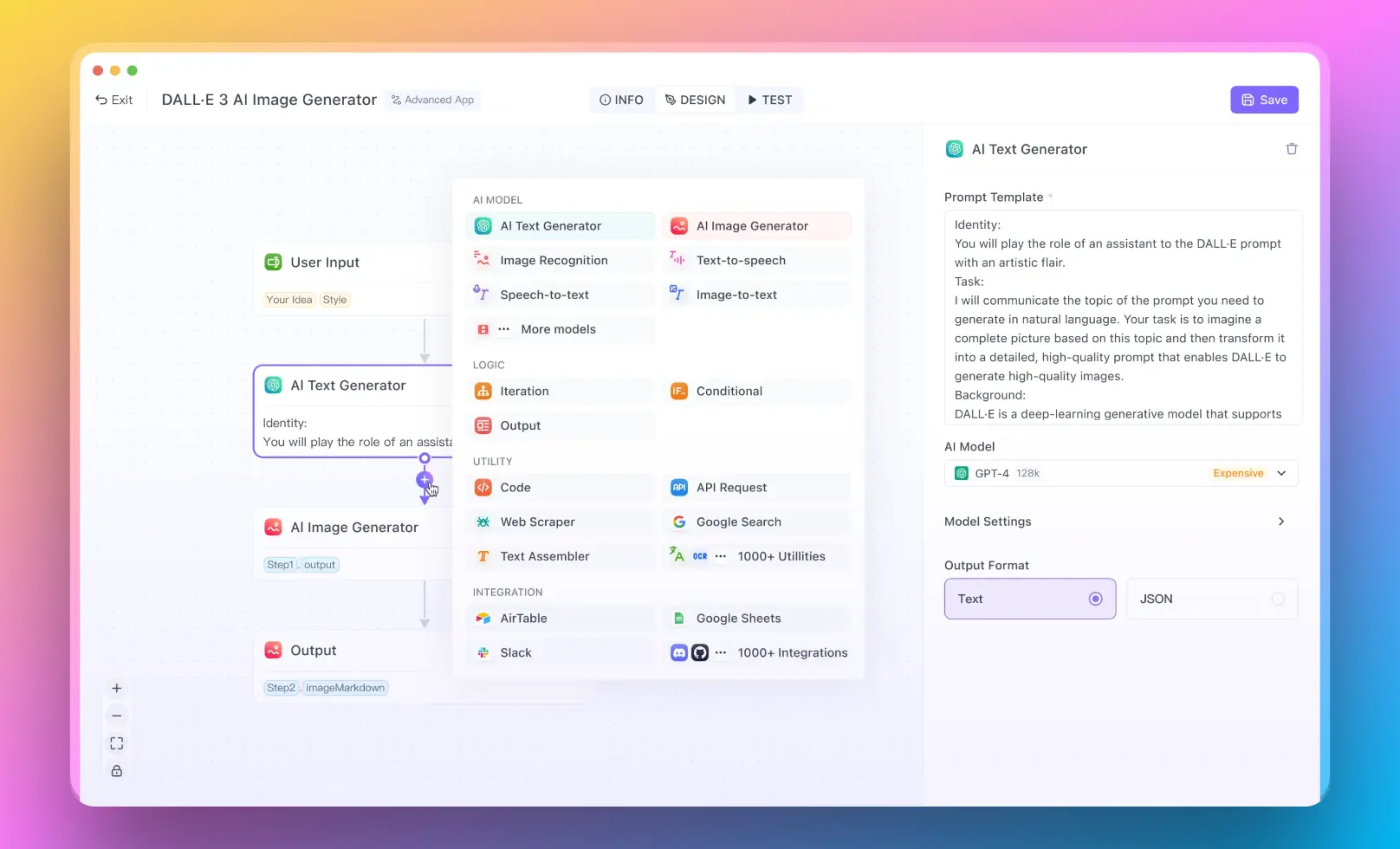
What is CSVChain in LangChain?
CSVChain is a module in the LangChain framework that enables you to easily load, parse, and interact with CSV (comma-separated values) files. It provides a convenient way to incorporate structured data stored in CSV format into your LangChain applications.
With CSVChain, you can:
- Read and parse CSV files
- Convert CSV data into vector representations
- Perform semantic search and question-answering over CSV data
- Integrate CSV data with other components of LangChain
Can LangChain Read CSV Files?
Yes, LangChain has built-in functionality to read and process CSV files using the CSVChain module. Here's a simple example of how to load a CSV file with CSVChain:
from langchain.chains import CSVChain
csv_path = "path/to/your/file.csv"
chain = CSVChain(csv_path=csv_path)
This code snippet creates a CSVChain
instance by specifying the path to your CSV file. LangChain will automatically read and parse the CSV data, making it accessible for further processing.
Using CSV Files in Vector Stores with LangChain
One powerful feature of CSVChain is its seamless integration with vector stores in LangChain. Vector stores allow you to convert your CSV data into high-dimensional vector representations, enabling efficient similarity search and retrieval.
Here's an example of how to use CSV files with a vector store in LangChain:
from langchain.vectorstores import FAISS
from langchain.embeddings import OpenAIEmbeddings
from langchain.chains import CSVChain
csv_path = "path/to/your/file.csv"
embeddings = OpenAIEmbeddings()
vector_store = FAISS.from_csv(csv_path, embeddings)
chain = CSVChain(vectorstore=vector_store)
In this code snippet:
- We import the necessary modules:
FAISS
for the vector store,OpenAIEmbeddings
for generating embeddings, andCSVChain
. - We specify the path to our CSV file.
- We create an instance of
OpenAIEmbeddings
to generate vector representations of our CSV data. - We create a
FAISS
vector store by callingfrom_csv()
and passing the CSV file path and embeddings. - Finally, we create a
CSVChain
instance, specifying the vector store.
With this setup, LangChain will automatically convert your CSV data into vector representations and store them in the FAISS vector store. You can then perform efficient similarity search and retrieval operations on your CSV data.
How the LangChain CSV Agent Works
The LangChain CSV agent is a powerful tool that allows you to interact with CSV data using natural language queries. It combines the capabilities of CSVChain with language models to provide a conversational interface for querying and analyzing CSV files.
Here's a high-level overview of how the LangChain CSV agent works:
CSV Data Loading:
- The agent starts by loading the specified CSV file using CSVChain.
- It parses the CSV data and extracts the relevant information.
Query Understanding:
- When you provide a natural language query to the agent, it uses a language model to understand the intent and extract the key information from the query.
- The agent analyzes the query to determine what kind of operation or analysis needs to be performed on the CSV data.
Data Retrieval and Processing:
- Based on the understood query, the agent retrieves the relevant data from the CSV file.
- It applies the necessary filtering, aggregation, or computation operations on the retrieved data.
- The agent may leverage vector stores or other LangChain components to perform advanced data processing tasks.
Response Generation:
- Once the agent has processed the data and obtained the results, it generates a natural language response.
- The response is crafted to provide the requested information or insights based on the CSV data.
- The agent may use language models or templating techniques to generate human-friendly responses.
Iterative Interaction:
- The LangChain CSV agent supports iterative interactions, allowing you to ask follow-up questions or provide additional instructions.
- It maintains the context of the conversation and can build upon previous queries and results.
Here's an example of how you can use the LangChain CSV agent:
from langchain.agents import create_csv_agent
from langchain.llms import OpenAI
csv_path = "path/to/your/file.csv"
agent = create_csv_agent(OpenAI(temperature=0), csv_path)
query = "What is the average price of products in the electronics category?"
response = agent.run(query)
print(response)
In this code snippet:
- We import the
create_csv_agent
function and theOpenAI
language model. - We specify the path to our CSV file.
- We create a CSV agent by calling
create_csv_agent()
and passing the language model and CSV file path. - We define a natural language query related to the CSV data.
- We run the query using the
run()
method of the agent and print the generated response.
The CSV agent will process the query, retrieve the relevant data from the CSV file, perform the necessary calculations (in this case, calculating the average price), and generate a natural language response with the requested information.
Understanding the CSV Quote_none Parameter
When working with CSVChain in LangChain, you may come across the quote_none
parameter. This parameter is used to specify how empty or missing values in the CSV file should be handled during parsing.
By default, when you create a CSVChain
instance, the quote_none
parameter is set to True
. This means that empty values in the CSV file will be treated as empty strings (""
) rather than the string "none"
.
Here's an example to illustrate the difference:
from langchain.chains import CSVChain
csv_path = "path/to/your/file.csv"
# Default behavior (quote_none=True)
chain_default = CSVChain(csv_path=csv_path)
# Explicitly set quote_none=False
chain_explicit = CSVChain(csv_path=csv_path, quote_none=False)
In the above code:
- When
quote_none
is set toTrue
(default behavior), empty values in the CSV file will be treated as empty strings (""
). - When
quote_none
is explicitly set toFalse
, empty values in the CSV file will be treated as the string"none"
.
The choice of setting quote_none
depends on your specific CSV file and how you want to handle empty or missing values. If your CSV file uses the string "none"
to represent empty values, you should set quote_none=False
. Otherwise, the default behavior (quote_none=True
) is usually sufficient.
By understanding the quote_none
parameter, you can ensure that your CSV data is parsed correctly and empty values are handled according to your requirements.
Conclusion
CSVChain and LangChain provide a powerful combination for working with CSV files and extracting insights from structured data. With CSVChain, you can easily read and parse CSV files, convert them into vector representations, and integrate them with other components of LangChain.
By leveraging the LangChain CSV agent, you can interact with your CSV data using natural language queries, allowing for intuitive data exploration and analysis. The agent understands your queries, retrieves relevant data from the CSV file, performs necessary processing, and generates human-friendly responses.
Whether you're a data scientist, analyst, or developer, CSVChain and LangChain offer a convenient and efficient way to work with CSV files and unlock the potential of your structured data. Start exploring the possibilities today and take your data analysis workflows to the next level!