When developing plugins in ChatGPT, you may come across the frustrating "TypeError: Failed to fetch" error, which occurs when making HTTP calls. Resolving this issue is vital for smooth plugin development. In this article, we will dive into the problem and provide comprehensive steps to solve it effectively.
What is the "Typeerror: Failed to Fetch Error"?
The "TypeError: Failed to fetch" error typically arises during local plugin development. It occurs when there is an issue with the HTTP request, preventing it from being successfully executed. This error can have multiple causes, including incorrect CORS (Cross-Origin Resource Sharing) settings or problems with network connectivity.
The "TypeError: Failed to fetch" error can manifest in various scenarios, leading to different error messages. Some common scenarios include:
- Making an API request to a different domain.
- Fetching resources from a local development server.
- Accessing data from an external API.
Here are a few examples of error messages users might encounter:
- "TypeError: Failed to fetch. Please check your network connection."
- "TypeError: Failed to fetch. The request was blocked due to CORS restrictions."
- "TypeError: Failed to fetch. The server responded with a 404 error."
Based on the details of your article and the GitHub thread you referenced, here are some additional points and sample code to enhance your article:
How to Solve the "Typeerror: Failed to Fetch Error"
Best Option: Develop with Anakin AI, a No Code AI App Buider
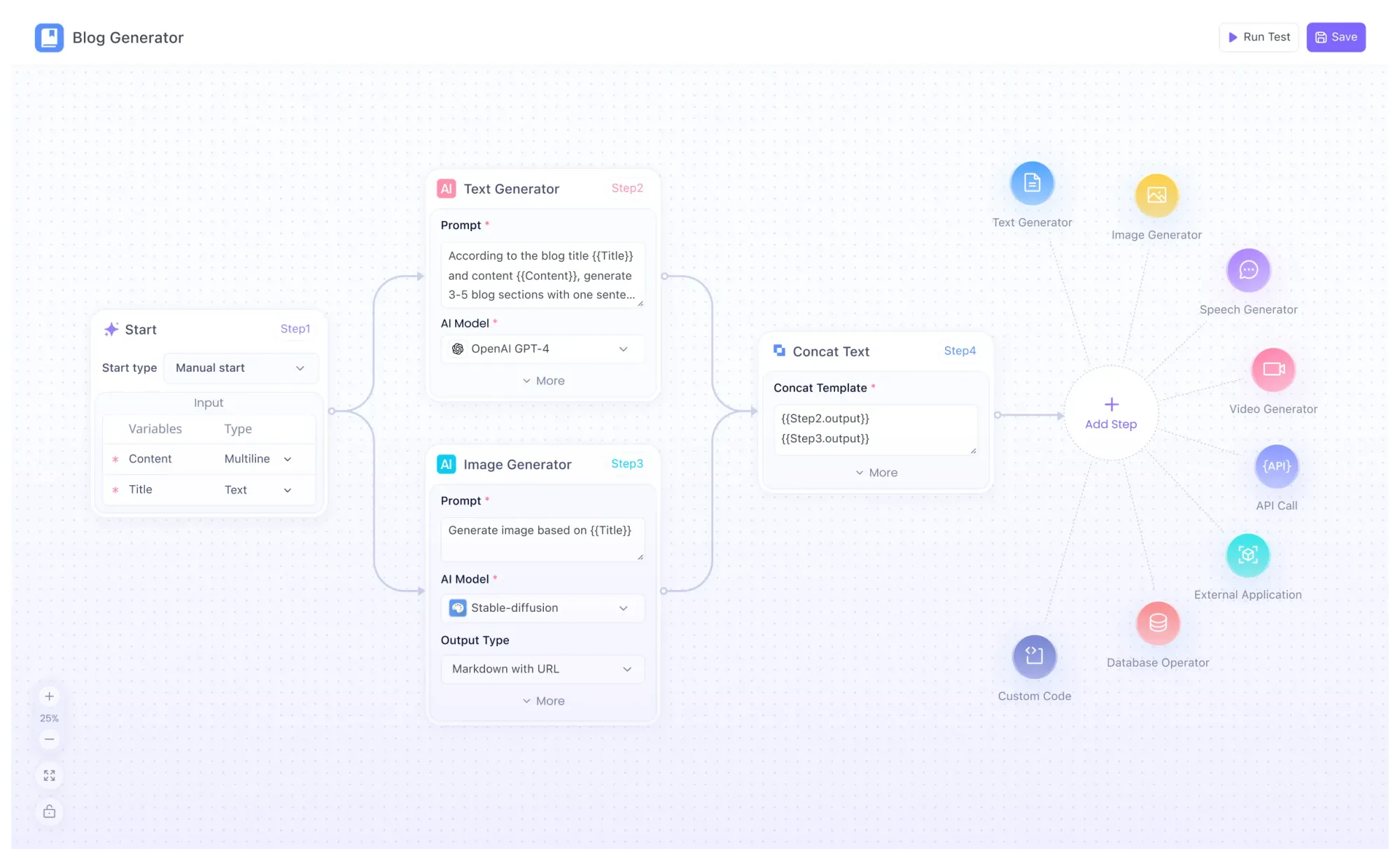
Anakin AI is a no-code platform that enables the creation of AI-powered applications using cutting-edge models such as GPT-4, Claude-2.1, Stable Diffusion, and DALL-E 3. This platform stands out for its user-friendly approach, allowing individuals and teams to efficiently build AI applications without the need for extensive coding knowledge.
Key Features of Anakin AI
- 1,000+ Pre-built AI Apps: Anakin AI hosts a vast library of specialized AI apps for various use cases like content generation, question answering, document search, and process automation. Users can select an app as it is or customize it to meet their specific needs.
- Diverse Application Range: The platform supports a wide array of applications including text generators, chatbots, image generators, workflow automations, batch processing, and auto agents.
- Custom AI App Development: Anakin AI’s no-code AI app builder allows users to create unique AI applications that automate tasks, generate content, answer questions, and more. This feature is designed to be user-friendly, enabling the creation of custom apps quickly and efficiently.
- Workflow and Batch Processing: These features significantly speed up various tasks, allowing for efficient handling of repetitive work. Users can automate content generation, data crawling, report production, and more without needing to learn coding or prompt configurations.
- Integration with External Tools and Services: Anakin AI offers seamless integration with existing tools and services, enhancing the functionality and adaptability of the AI applications created on the platform.
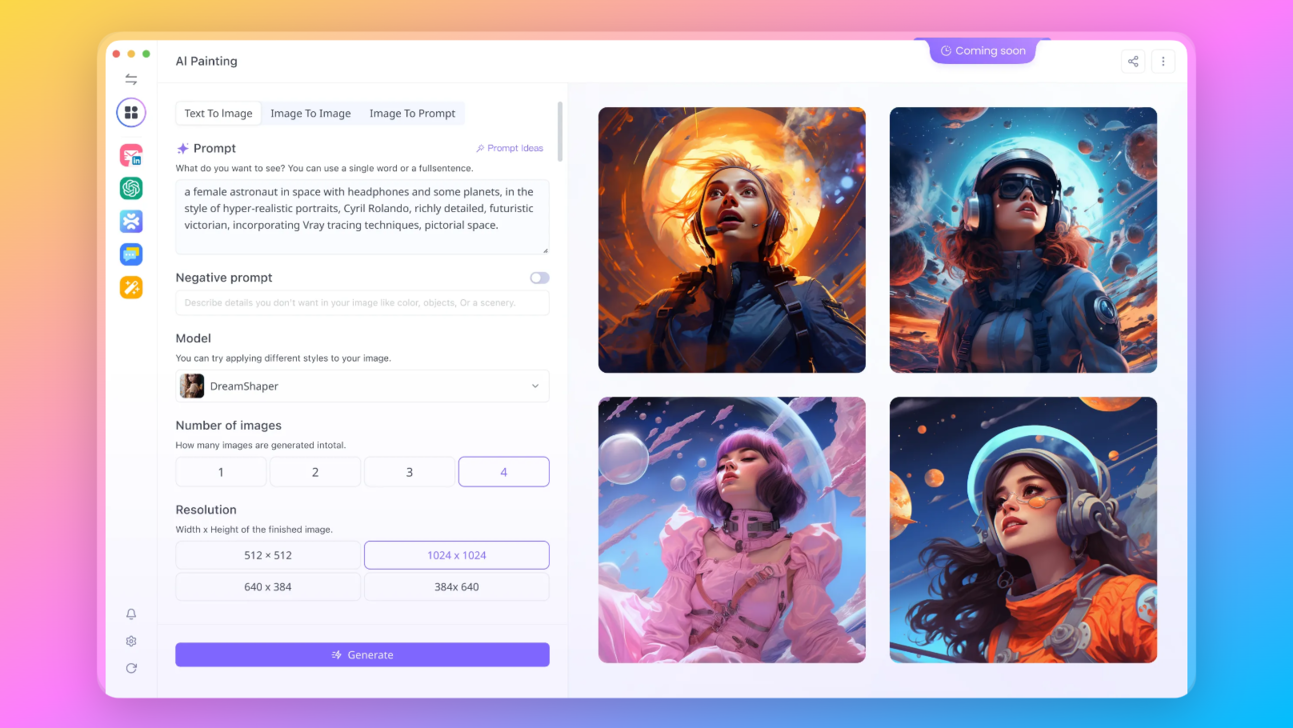
Interested? Try Anakin AI right now!👇👇👇
Solutions to "Typeerror: Failed to Fetch Error" of OpenAI API
Using Proxies: If the "TypeError: Failed to fetch" error is due to geo-restrictions (like the OpenAI API ban in China), using a proxy can be an effective solution. Implement a proxy in your code to route your requests through a different geographical location.
Sample Code:
const fetch = require('node-fetch');
const HttpsProxyAgent = require('https-proxy-agent');
const proxyAgent = new HttpsProxyAgent('http://your-proxy-address:port');
fetch(url, { agent: proxyAgent })
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
VPN Usage: For individual developers, using a VPN can change your IP address, potentially bypassing the geo-restriction. This is a straightforward approach but might not be feasible in a production environment.
Alternative APIs: If specific APIs are not accessible due to regional bans, explore using alternative APIs that offer similar functionalities but are not subject to the same restrictions.
Best Practics to Avoid the "Typeerror: Failed to Fetch Error" Error
Using HTTPS: Ensure that your requests are made over HTTPS, as many APIs do not support unencrypted HTTP requests.
Regularly Review Dependencies: Keep your libraries and dependencies up-to-date to ensure compatibility with external APIs and to benefit from the latest security patches.
Environment-Specific Configuration: For applications deployed in multiple environments (development, staging, production), ensure CORS and proxy settings are appropriately configured for each environment.
Here are the sample codes to potentially sovle the issue:
Django CORS Issue Solution:
# Install django-cors-headers
pip install django-cors-headers
# In settings.py
INSTALLED_APPS = [
...
'corsheaders',
...
]
MIDDLEWARE = [
...
'corsheaders.middleware.CorsMiddleware',
...
]
# Allow all origins (not recommended for production)
CORS_ALLOW_ALL_ORIGINS = True
Node.js Solution Using Axios and Proxy:
const axios = require('axios');
const HttpsProxyAgent = require('https-proxy-agent');
axios({
method: 'get',
url: 'https://api.example.com/data',
proxy: false,
httpsAgent: new HttpsProxyAgent('http://your-proxy-address:port')
})
.then(response => console.log(response.data))
.catch(error => console.error('Error:', error));
FAQs
What are the implications of using a proxy for API requests?
Using a proxy can add an additional layer of complexity and might introduce latency. It is crucial to choose a reliable and fast proxy to minimize these effects.
Can CORS settings be dynamically adjusted in my application?
Yes, in many server-side applications, you can dynamically adjust CORS settings based on the environment or other criteria. However, this should be done cautiously to avoid introducing security vulnerabilities.
Are there any tools to test CORS issues locally?
Tools like Postman or browser developer tools can be used to test and debug CORS issues. They provide detailed information about the requests and responses, including headers that can help in diagnosing CORS problems.
Conclusion
The "TypeError: Failed to fetch" error can be a frustrating obstacle during plugin development in ChatGPT. By following the troubleshooting steps and implementing best practices outlined in this article, you can effectively solve the issue and enjoy a smoother development experience.