Introduction: What's the Buzz About Apple's MLX?
Have you heard about MLX? It's the latest buzz in the tech world, especially among those keen on machine learning and Apple's ecosystem. But why are developers and tech enthusiasts so excited about it? Let's dive in and find out.
Why MLX, and why now? In the realm of machine learning, having tools that seamlessly integrate with your hardware is a game-changer. Apple's MLX, an advanced machine learning framework, is designed precisely with this in mind. It stands out for its ability to harness the full potential of Apple’s cutting-edge silicon, including the A-series and M-series chips. This framework isn't just another tool; it's a significant leap forward in machine learning capabilities for Apple devices.
So, what can MLX really do? Imagine having a toolbox that not only fits perfectly in your hand but also multiplies your capabilities manifold. That's MLX for you - offering Python and C++ APIs similar to NumPy and PyTorch, it provides an intuitive and powerful platform for developers. Whether you're a seasoned coder or just starting, MLX is designed to make your journey in machine learning on Apple devices smoother, more efficient.
Want to access GPT-4, but having trouble registering a ChatGPT Plus Account?
Visit Anakin AI right now to access GPT-4 models with No Waiting Time!
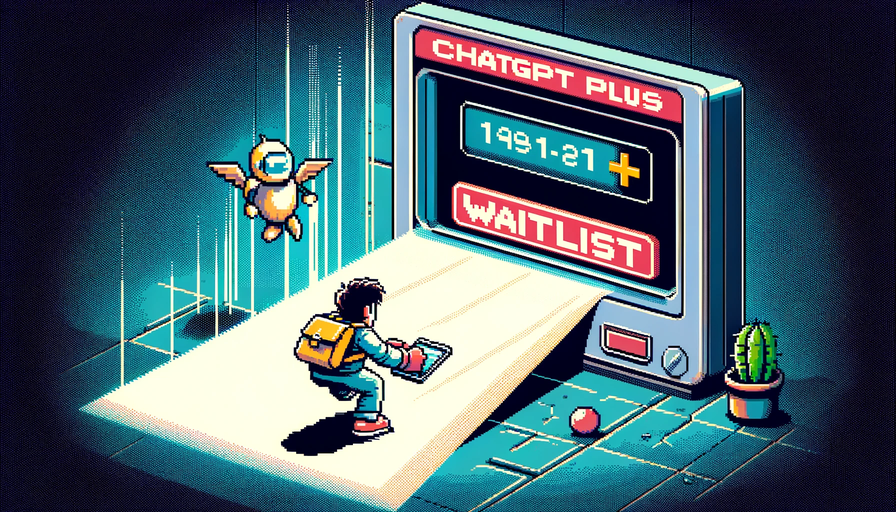
Key Features of Apple's MLX: Why Should You Care?
What makes MLX stand out in the crowded space of machine learning frameworks? Well, it's all about optimization and familiarity. Let’s break it down:
- Familiar APIs: For developers who have worked with Python and PyTorch, MLX feels like a familiar friend. It provides similar APIs, making the transition smoother and the learning curve gentler.
- Optimized for Apple Hardware: The real magic of MLX lies in its optimization for Apple hardware. It leverages the power of Apple's A-series and M-series chips, promising enhanced performance for both iOS and iPadOS applications.
But is optimization really that big a deal? Absolutely! In the world of machine learning, performance is key. The faster and more efficiently you can train and run your models, the quicker you can iterate and improve. MLX's optimization ensures that developers can make the most out of Apple's powerful hardware, leading to faster, more efficient machine learning applications.
Let’s talk real-world impact: Imagine you're building a complex machine learning model for an iOS app. With MLX, you can expect smoother running models, quicker training times, and overall a more seamless experience both for the developer and the end-user. It’s not just about building models; it’s about building them better, faster.
How to Install MLX on M1/M2 Mac
So, how easy is it to get started with MLX? This is often the first stumbling block for many when exploring new tech. Thankfully, MLX doesn't disappoint. The installation process is straightforward, but let's dig a little deeper.
Installation: First, ensure you have the prerequisites in place. This includes having a compatible Apple device and the necessary software environment. Then simply run this command to install MLX:
pip install mlx
And that's it!
But what about after installation? Once MLX is installed, the next step is to dive into its capabilities. The quick start guide provides a clear path for beginners. It's like having a friendly guide by your side, leading you through the initial steps of using MLX.
import mlx
# Initialize MLX for your specific application
mlx.init()
Why is a good start crucial? A smooth start sets the tone for your entire journey with a new tool. The quick start guide for MLX ensures that you're not left guessing about what to do next. It's about building confidence as you take your first steps in this new environment.
How to Use Apple's MLX for Machine Learning Tasks
Thank you for providing the detailed code examples. Incorporating these into the article will greatly enhance its practical value and depth. Below, I'll integrate these code snippets into the relevant sections of the article, ensuring they are well-explained and contribute effectively to the overall narrative.
Linear Regression with Apple's MLX: A Practical Walkthrough
How do we implement a basic linear regression model with MLX? Let's start with a hands-on example to understand the mechanics of MLX. We begin by setting up some essential parameters:
import mlx.core as mx
num_features = 100
num_examples = 1_000
num_iters = 10_000 # iterations of SGD
lr = 0.01 # learning rate for SGD
But what about the data? Here's how we generate a synthetic dataset:
- Sample the design matrix
X
. - Create a ground truth parameter vector
w_star
. - Compute the dependent values
y
by adding Gaussian noise toX @ w_star
.
# True parameters
w_star = mx.random.normal((num_features,))
# Input examples (design matrix)
X = mx.random.normal((num_examples, num_features))
# Noisy labels
eps = 1e-2 * mx.random.normal((num_examples,))
y = X @ w_star + eps
What's next? The optimization step. We use Stochastic Gradient Descent (SGD) to find the optimal weights:
def loss_fn(w):
return 0.5 * mx.mean(mx.square(X @ w - y))
grad_fn = mx.grad(loss_fn)
w = 1e-2 * mx.random.normal((num_features,))
for _ in range(num_iters):
grad = grad_fn(w)
w = w - lr * grad
mx.eval(w)
How do we verify our results? By computing the loss of the learned parameters and comparing them to the ground truth:
loss = loss_fn(w)
error_norm = mx.sum(mx.square(w - w_star)).item() ** 0.5
print(f"Loss {loss.item():.5f}, |w-w*| = {error_norm:.5f}")
# Expected output: Loss 0.00005, |w-w*| = 0.00364
Building a Multi-Layer Perceptron with Apple's MLX: Step by Step
Want to go a step further? Let's tackle a multi-layer perceptron to classify MNIST using mlx.nn
:
Import the necessary MLX packages:
import mlx.core as mx
import mlx.nn as nn
import mlx.optimizers as optim
import numpy as np
Define the MLP class by extending mlx.nn.Module
:
class MLP(nn.Module):
def __init__(
self, num_layers: int, input_dim: int, hidden_dim: int, output_dim: int
):
super().__init__()
layer_sizes = [input_dim] + [hidden_dim] * num_layers + [output_dim]
self.layers = [
nn.Linear(idim, odim)
for idim, odim in zip(layer_sizes[:-1], layer_sizes[1:])
]
def __call__(self, x):
for l in self.layers[:-1]:
x = mx.maximum(l(x), 0.0)
return self.layers[-1](x)
Define the loss function and an evaluation function:
def loss_fn(model, X, y):
return mx.mean(nn.losses.cross_entropy(model(X), y))
def eval_fn(model, X, y):
return mx.mean(mx.argmax(model(X), axis=1) == y)
Set up the problem parameters and load the data:
num_layers = 2
hidden_dim = 32
num_classes = 10
batch_size = 256
num_epochs = 10
learning_rate = 1e-1
# Load the data
import mnist
train_images, train_labels, test_images, test_labels = map(
mx.array, mnist.mnist()
)
Create a batch iterator for SGD and run the training loop:
def batch_iterate(batch_size, X, y):
perm = mx.array(np.random.permutation(y.size))
for s in range(0, y.size, batch_size):
ids = perm[s : s + batch_size]
yield X[ids], y[ids]
model = MLP(num_layers, train_images.shape[-1], hidden_dim, num_classes
)
mx.eval(model.parameters())
loss_and_grad_fn = nn.value_and_grad(model, loss_fn)
optimizer = optim.SGD(learning_rate=learning_rate)
for e in range(num_epochs):
for X, y in batch_iterate(batch_size, train_images, train_labels):
loss, grads = loss_and_grad_fn(model, X, y)
optimizer.update(model, grads)
mx.eval(model.parameters(), optimizer.state)
accuracy = eval_fn(model, test_images, test_labels)
print(f"Epoch {e}: Test accuracy {accuracy.item():.3f}")
```
LLM Inference with Apple's MLX
What about large transformers on Apple silicon? MLX makes it efficient and straightforward. Let's look at an example script for Llama transformer models:
Start with implementing the Attention layer and Encoder layer:
# Attention layer
class LlamaAttention(nn.Module):
# ... implementation details ...
# Encoder layer
class LlamaEncoderLayer(nn.Module):
# ... implementation details ...
Define the full model by combining LlamaEncoderLayer instances:
class Llama(nn.Module):
# ... implementation details ...
Implement the generation function for inference:
class Llama(nn.Module):
# ... previous code ...
def generate(self, x, temp=1.0):
# ... implementation details ...
Create and use the Llama model for sampling tokens:
model = Llama(num_layers=12, vocab_size=8192, dims=512, mlp_dims=1024, num_heads=8)
mx.eval(model.parameters())
prompt = mx.array([[1, 10, 8, 32, 44, 7]])
generated = [t for i, t in zip(range(10), model.generate(prompt, 0.8))]
mx.eval(generated)
Incorporating these examples into the article provides readers with practical insights into using MLX for various machine learning tasks. It demonstrates the framework's versatility and ease of use, particularly on Apple hardware. This practical approach will make the article more engaging and valuable for the target audience.
Utilizing Streams in Apple's MLX: What's the Big Deal?
Now, what about using streams in MLX? Streams are a powerful feature in MLX, but what makes them so special? Let's find out.
- Stream Functionality Explained: In simple terms, streams in MLX allow for more efficient data processing. They enable parallel operations, making your machine learning tasks run faster and more smoothly.
But how does this impact your work? Imagine you're juggling multiple tasks simultaneously. Without streams, these tasks might bottleneck, slowing down your workflow. With streams, you can handle multiple tasks concurrently, boosting your efficiency.
with mlx.stream():
# Your machine learning code goes here
pass
Is it just about speed? Speed is a significant factor, but it's not the only benefit. Streams also allow for better resource management, ensuring that your machine learning models are not just fast, but also resource-efficient.
And what about more complex tasks? MLX isn't limited to simple tasks. It shines in handling more complex scenarios, like the llama inference example. This shows MLX's capability in dealing with intricate machine learning models.
Does MLX support innovative approaches? Absolutely! The potential Swift frontend for iOS/iPadOS indicates MLX's adaptability and forward-thinking approach. It’s about staying ahead in the game and making machine learning more accessible and efficient on Apple devices.
You can check out the Apple's MLX Github page here.
Conclusion: The Bright Future of Apple's MLX in Machine Learning
So, what have we learned about MLX? MLX is more than just a new entry in the machine learning landscape; it's a significant step forward for those invested in the Apple ecosystem. It marries the power of Apple's hardware with the flexibility and familiarity of popular programming languages.
- Unleashing the Power of Apple Hardware: MLX's biggest draw is its optimization for Apple's hardware. This optimization means that whether you're working on complex algorithms or simple data processing tasks, you can expect improved performance, efficiency, and speed.
- A Familiar Playground for Developers: With APIs similar to NumPy and PyTorch, MLX offers a comfortable environment for developers. This familiarity reduces the learning curve, making it easier for new users to adopt and for seasoned professionals to transition their skills.
But what does the future hold for MLX? The future of MLX looks promising. Its current capabilities already position it as a powerful tool for machine learning on Apple devices. However, the potential for growth and improvement is vast.
- Anticipating Future Enhancements: We can expect regular updates and enhancements to MLX, keeping it in step with the evolving needs of machine learning and Apple's hardware advancements.
- Expanding the Horizons of MLX: There's also the potential for expanding its capabilities beyond current offerings. This could include better integration with other Apple services, enhanced support for more complex machine learning models, and perhaps even more streamlined processes for deploying MLX-based models in real-world applications.
Conclusion
In conclusion, Apple's MLX is not just a tool for today; it's an investment in the future of machine learning on Apple devices. It offers the perfect blend of power, efficiency, and user-friendliness, making it an attractive option for anyone looking to explore machine learning in the Apple ecosystem. As MLX continues to evolve, we can only imagine the possibilities it will unlock for developers and innovators around the world.
Want to access GPT-4, but having trouble registering a ChatGPT Plus Account?
Visit Anakin AI right now to access GPT-4 models with No Waiting Time!
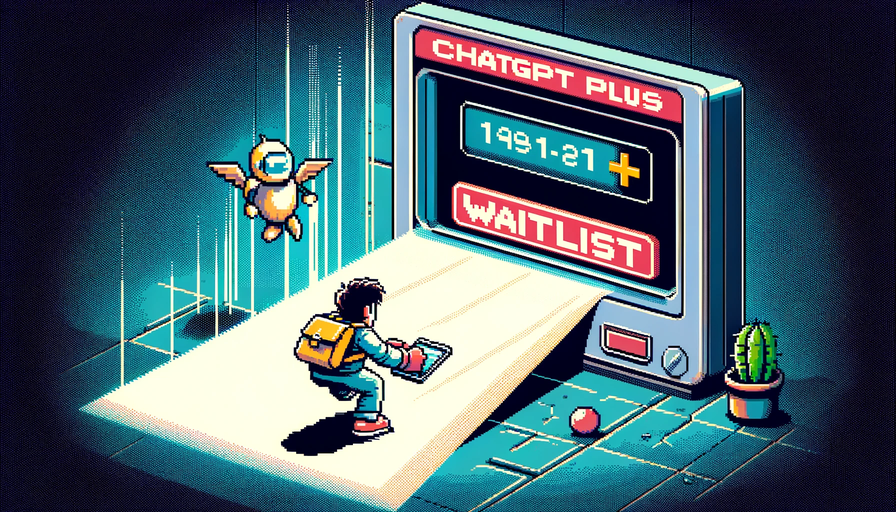