Welcome to the pragmatic world of Python programming with OpenAI API!
In this article, we dive into the most common hiccups you might face, from the perplexing 'choices' attribute challenges to the elusive 'ChatCompletion' attribute. It's a journey through the twists and turns of API responses and prompt structures!
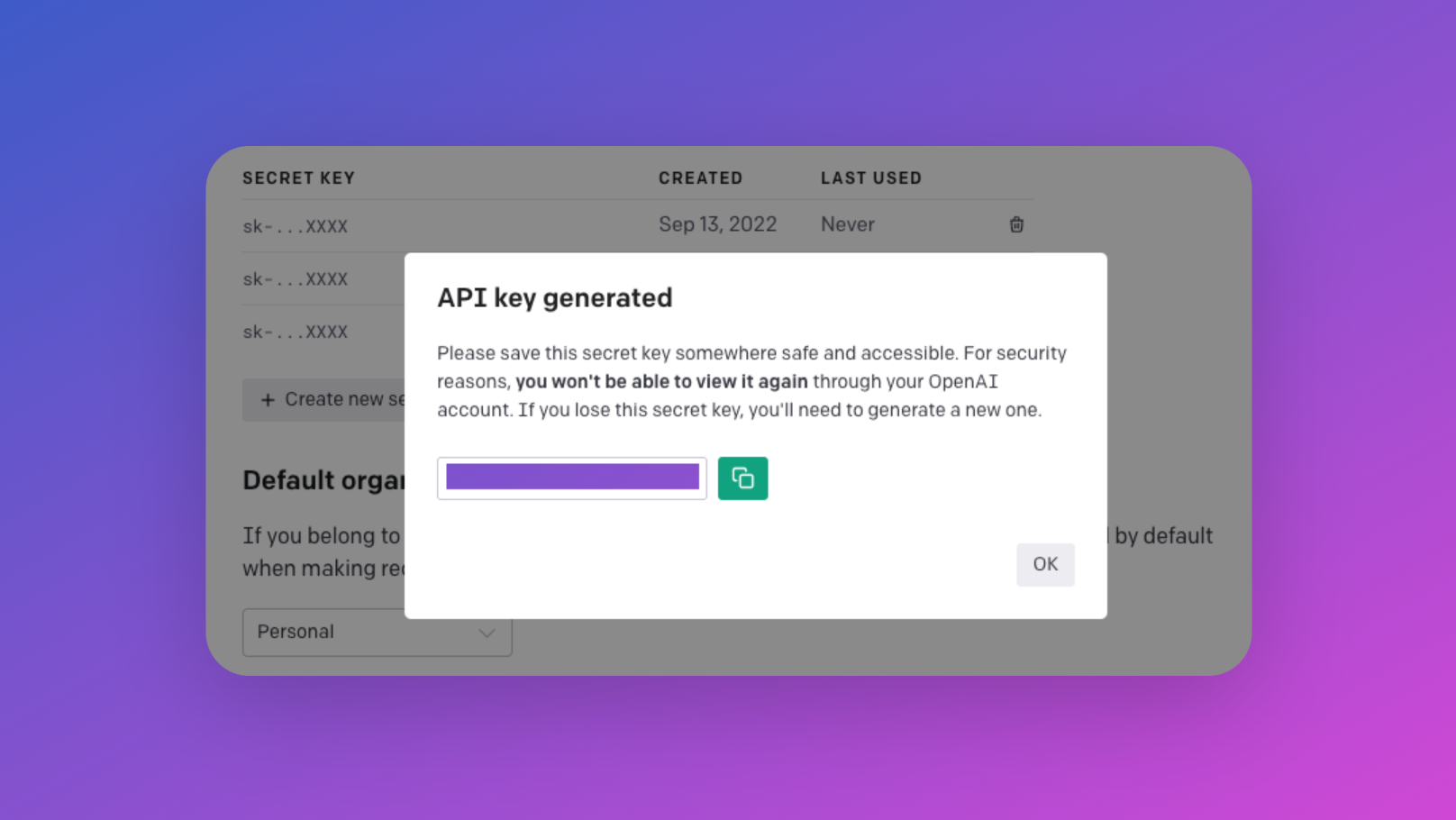
Perfect for both the seasoned coder and the curious newcomer, weโre tackling these issues head-on, armed with practical solutions and a dash of coding savvy. Get ready to conquer these hurdles and smooth out your OpenAI API experience!
Keypoints Covered in This Article
This article addresses key concerns like:
- TyperError with the choices attribute
- TypeError: Cannot read properties of undefined (reading 'choices')
- KeyError: 'choices'
- Empty responses in OpenAI API
- Fixing 'No attribute ChatCompletion' errors.
Let's dive in!
Want to try out a No Code Platform to build AI Apps?
No need to look around, Anakin AI got you covered!
You can effortless build any customized AI App within 5 minutes, without any issue in coding errors!
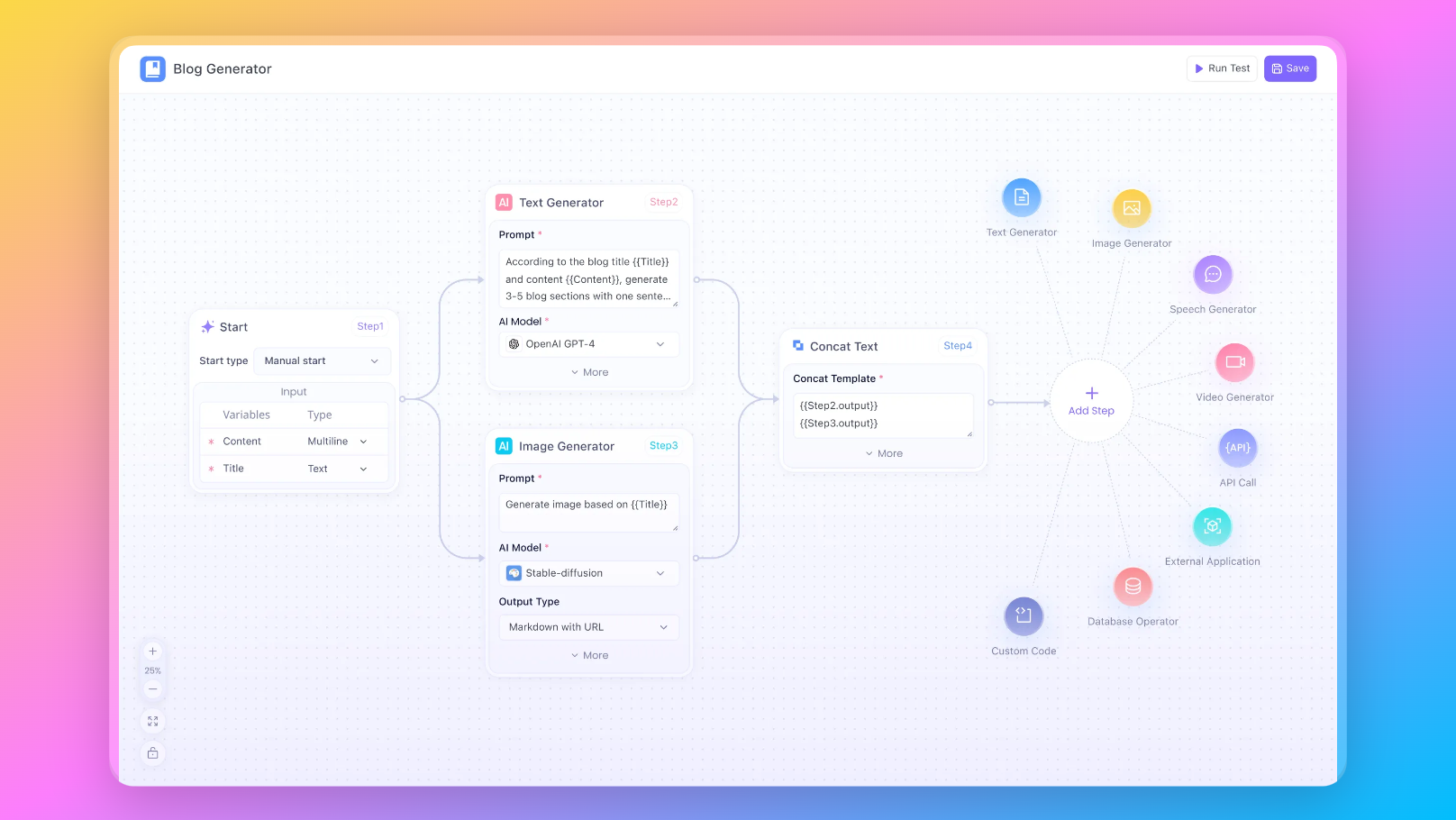
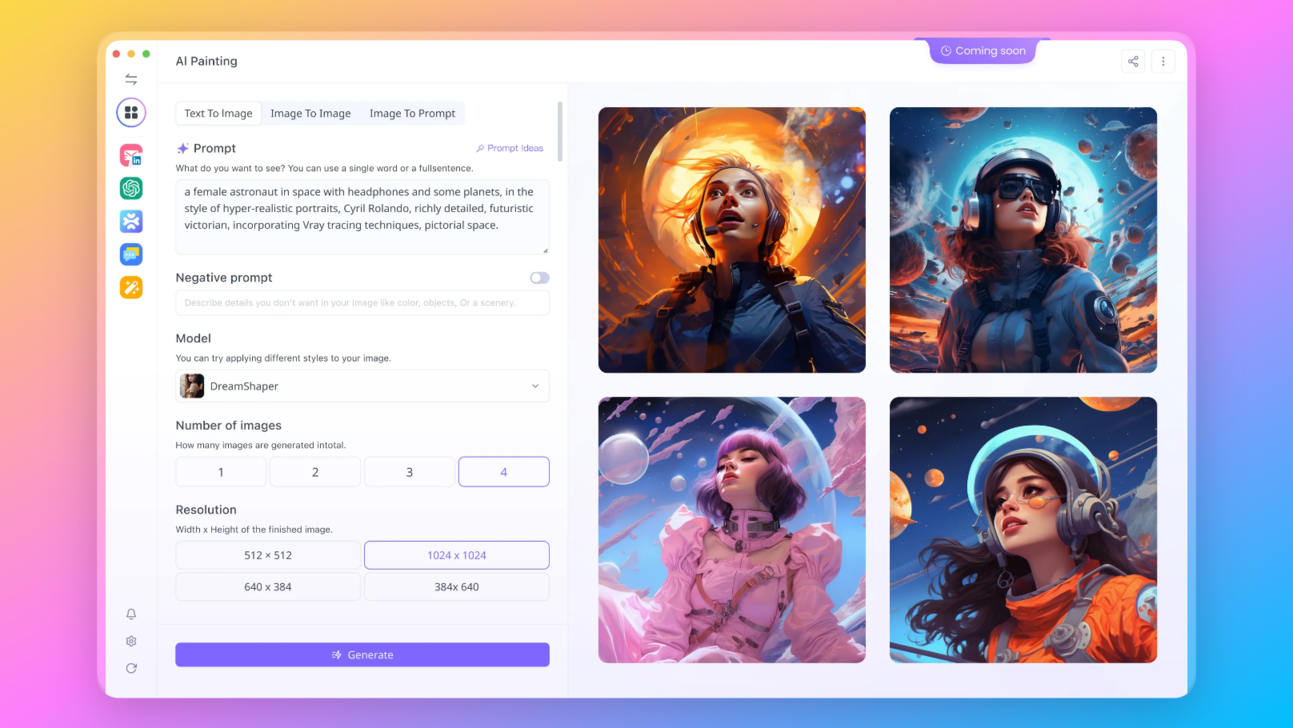
Interested? Check out Anakin AI right not for free!๐๐๐
1. How to Fix TypeError For 'choices' Attribute with OpenAI API (in Python)
Issue: Users face a TypeError
when accessing the 'choices' attribute in the OpenAI API response, hinting at an environmental or syntax issue.
Reasons:
- Environment Issues: Possible outdated or conflicting Python or OpenAI library versions.
- Syntax Errors: Incorrect code syntax for accessing 'choices'.
- API Response Misinterpretation: Misunderstanding the structure of the API response.
Solution:
- Reinstall Python and Libraries: Ensuring a clean and updated Python environment can resolve underlying compatibility issues.
pip install --upgrade python openai
- Check Syntax and Response Handling:
- Carefully review the code for syntax accuracy, particularly when accessing the 'choices' attribute.
- Understand the structure of the API response to correctly extract data.
- Regular testing and reviewing the API documentation can prevent and resolve such issues.
2. How to Solve 'TypeError: Cannot read properties of undefined (reading 'choices')` in Node.js
Issue:
Developers encounter a TypeError: Cannot read properties of undefined (reading 'choices')
in Node.js when accessing the 'choices' attribute.
Reasons:
- Misconfiguration of API Key: Not setting the API key correctly in the environment.
- Improper Response Handling: Failing to manage the API response accurately.
- Syntax Errors: Mistakes in the code syntax while accessing response properties.
Solution:
- Ensure Proper API Key Configuration: Verify that the API key is correctly set and accessible in the Node.js environment.
require('dotenv').config();
const openai = new OpenAI({ apiKey: process.env.OPENAI_API_KEY });
- Handle API Responses Accurately: Log the entire API response and check if the expected data exists before trying to access it.
const response = await openai.chat.completions.create({ ... });
console.log('API Response: ', response);
if (response && response.data && response.data.choices && response.data.choices.length > 0) {
let content = response.data.choices[0].message.content;
console.log('Response content: ', content);
} else {
throw new Error('Unexpected API response format.');
}
- Debug and Refine Code: Review and refine the code to ensure correct syntax and logic, and handle any potential errors effectively.
3. How to Handle KeyError: 'choices' Attribute Errors of OpenAI API (in Python)
Issue:
New Python developers sometimes face a KeyError: 'choices'
when trying to access the 'choices' attribute, indicating that the key is not found in the response dictionary.
Reasons:
- Syntax Error: Typographical mistakes in the code.
- Misunderstanding API Response: Not fully understanding the structure of the API response.
- Incomplete API Call: The API call is not formed correctly, resulting in an incomplete response.
Solution:
- Correct Syntax: Check for any syntax errors in the code.
import openai
response = openai.Completion.create(...)
2. Understand Response Structure: Ensure understanding of the response's format.
print(response)
3. Complete API Call: Verify that the API call includes all necessary parameters.
response = openai.Completion.create(prompt="Hello, world!", engine="davinci", max_tokens=50)
4. How to Solve Empty Responses in ChatGPT API with Python
Issue:
Users receive empty responses from the ChatGPT API, such as {}
or {'choices': []}
, especially with lengthy or complex prompts.
Reasons:
- Exceeding Token Limit: The prompt's length surpasses the API's token limit.
- Prompt Structure: Complex or improperly structured prompts leading to no response.
Solution:
- Adjust Prompt Length: Shorten the prompt to stay within the token limit.
prompt = "Short and concise prompt"
- Refine Prompt Structure: Simplify the prompt's structure for clarity.
response = openai.Completion.create(prompt=prompt, max_tokens=150)
5. How to Fix 'No attribute ChatCompletion' Error for OpenAI API in Python
Issue: Users encounter an error stating, "AttributeError: module 'openai' has no attribute 'ChatCompletion'", indicating an issue with library setup or usage.
Reasons:
- Outdated Library Version: Using an older version of the OpenAI library.
- Incorrect Python Version: Not using a compatible version of Python.
- Installation Issues: Problems with the library installation.
Solution:
- Update OpenAI Library: Make sure the latest version of the OpenAI library is installed.
pip install --upgrade openai
- Check Python Version: Ensure that a compatible version of Python is being used.
- Reinstall Library: If necessary, reinstall the OpenAI library in a clean environment.
pip uninstall openai
pip install openai
Want to try out a No Code Platform to build AI Apps?
No need to look around, Anakin AI got you covered!
You can effortless build any customized AI App within 5 minutes, without any issue in coding errors!
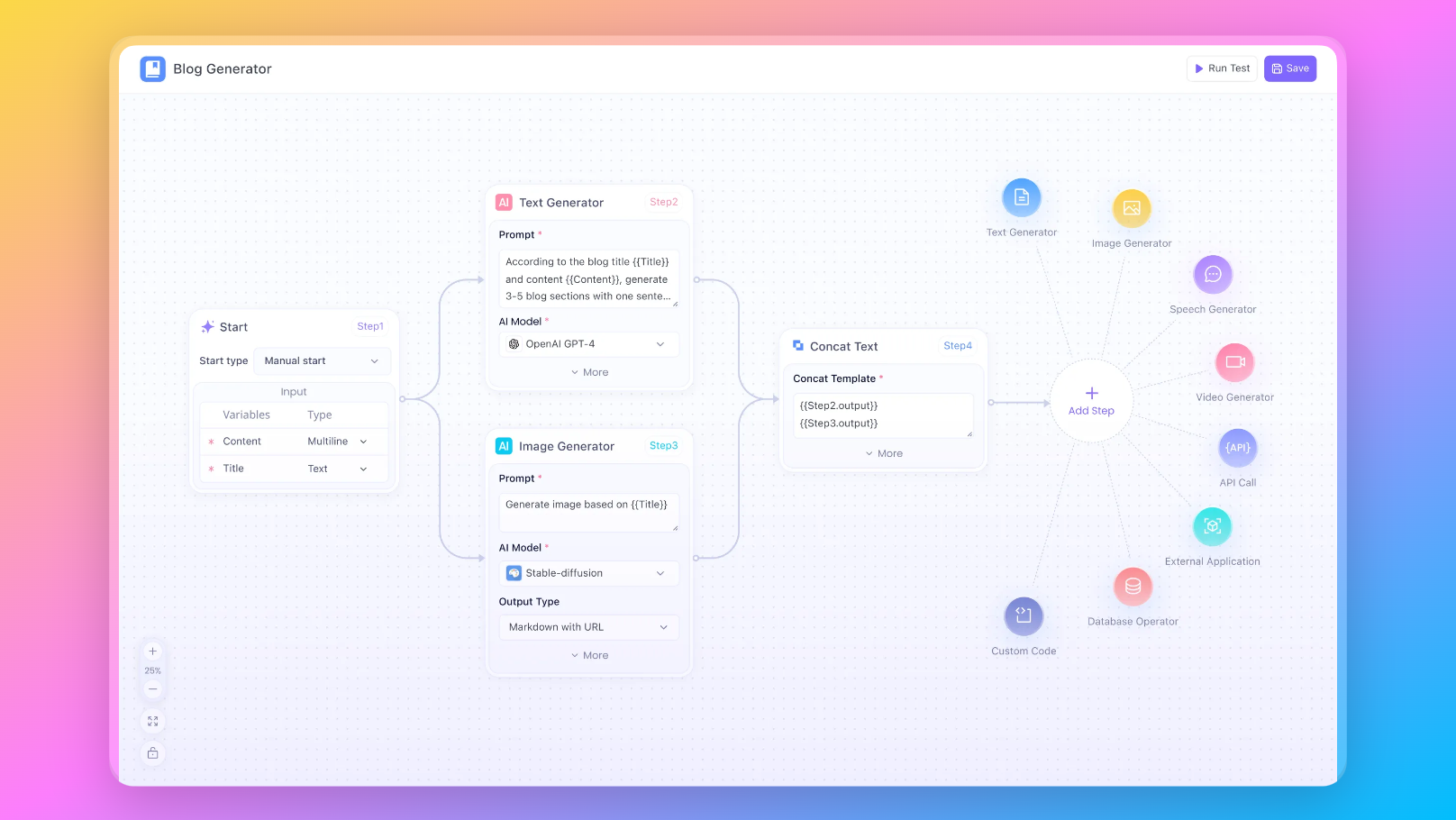
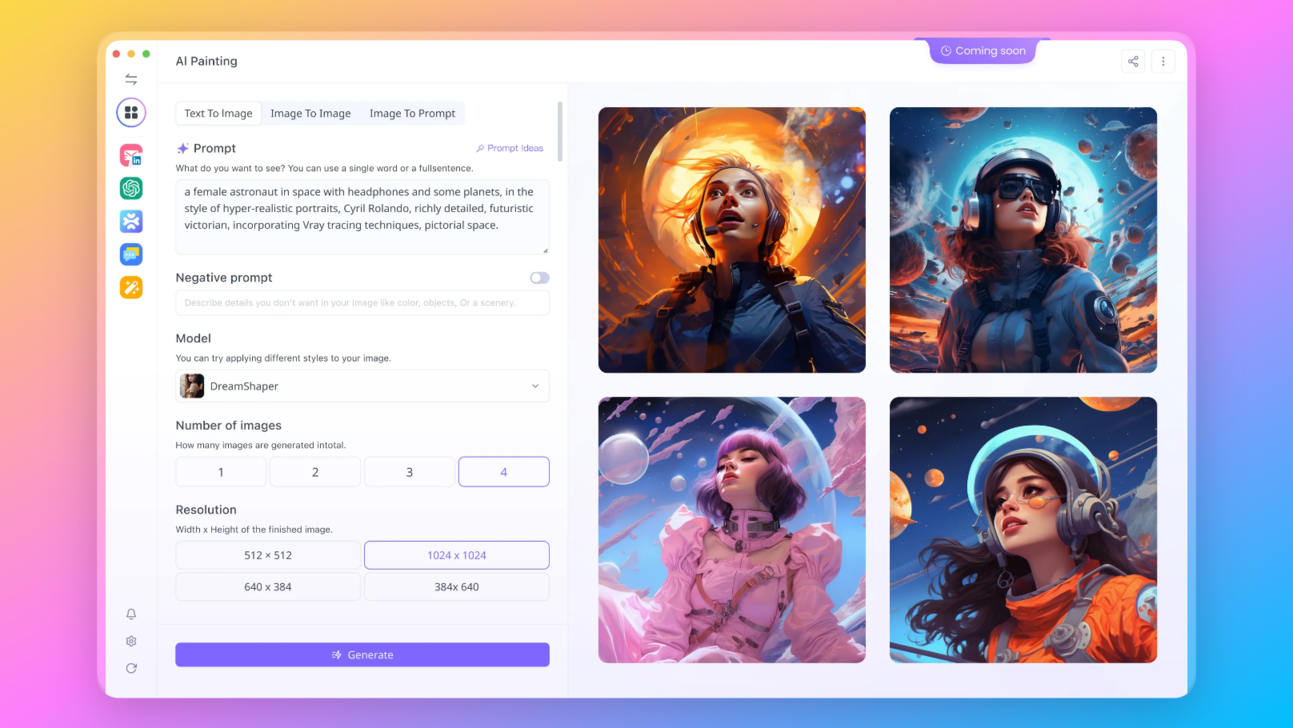
Interested? Check out Anakin AI right not for free!๐๐๐
Conclusion
Mastering these solutions to the top OpenAI API issues can significantly enhance your programming experience. Whether it's navigating 'choices' attribute errors, tackling empty OpenAI API responses, or fixing attribute errors, these insights are invaluable. Embrace these strategies to optimize your use of OpenAI's powerful API.