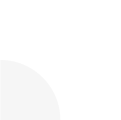

Regex by Example | Free AI tool
A better way to generate regex when you have an example; And it works for multiline input like csv or long text; Optional generate code like js, ts, java etc.
Introduction
A Better Regex Generator
Give AI an example and tell AI what you want, then generate code in which language you selected.
You can run the code immediately!
Works with CSV Input
You can use csv as input, just like:
Year,Make,Model,Description,Price
1997,Ford,E350,"ac, abs, moon",3000.00
1999,Chevy,"Venture ""Extended Edition""","",4900.00
1999,Chevy,"Venture ""Extended Edition, Very Large""","",5000.00
1996,Jeep,Grand Cherokee,"MUST SELL!
air, moon roof, loaded",4799.00
And tell AI what you want:
the model cheaper than 4800
Then you can get the regex and code:
const regex = /[^,\n]+,[^,\n]+,[^,\n]+,[^,\n]+,(\d+\.\d+)/g;
const matchModelCheaperThan4800 = [];
let match;
while ((match = regex.exec(data)) !== null) {
if (parseFloat(match[1]) < 4800) {
matchModelCheaperThan4800.push(match[0]);
}
}
console.log(matchModelCheaperThan4800);
Support Different Language
I had add 5 languages:
- JaveScript
- TypeScript
- C/C++
- JAVA
- Rust
Don't be afraid that the language you used is not supported, just say to AI:
Give me an kotlin example.
then you can get the code in your language
fun main() {
val data = """
1997,Ford,E350,"ac, abs, moon",3000.00
1999,Chevy,"Venture ""Extended Edition""","",4900.00
1999,Chevy,"Venture ""Extended Edition, Very Large""","",5000.00
1996,Jeep,Grand Cherokee,"MUST SELL!
air, moon roof, loaded",4799.00
""".trimIndent()
val regex = Regex("[^,\n]+,[^,\n]+,[^,\n]+,[^,\n]+,(\\d+\\.\\d+)")
val matchModelCheaperThan4800 = mutableListOf<String>()
regex.findAll(data).forEach {
if (it.groupValues[1].toFloat() < 4800) {
matchModelCheaperThan4800.add(it.value)
}
}
for (match in matchModelCheaperThan4800) {
println(match)
}
}
It works!
Prompt Template
You are a senior programmer, and I have some regex question. Please give me an regular expression that can match {{What you want to match}} in:```{{Long Text or any input}}``` And better way is give me some example code in {{Program Language}}