Ollama is a powerful tool that allows you to run large language models (LLMs) locally on your machine. This article will guide you through the process of setting up, configuring, and using Ollama for various applications. We'll cover everything from installation to advanced usage, including code examples to help you get started quickly.
Then, You cannot miss out Anakin AI!
Anakin AI is an all-in-one platform for all your workflow automation, create powerful AI App with an easy-to-use No Code App Builder, with Llama 3, Claude Sonnet 3.5, GPT-4, Uncensored LLMs, Stable Diffusion...
Build Your Dream AI App within minutes, not weeks with Anakin AI!
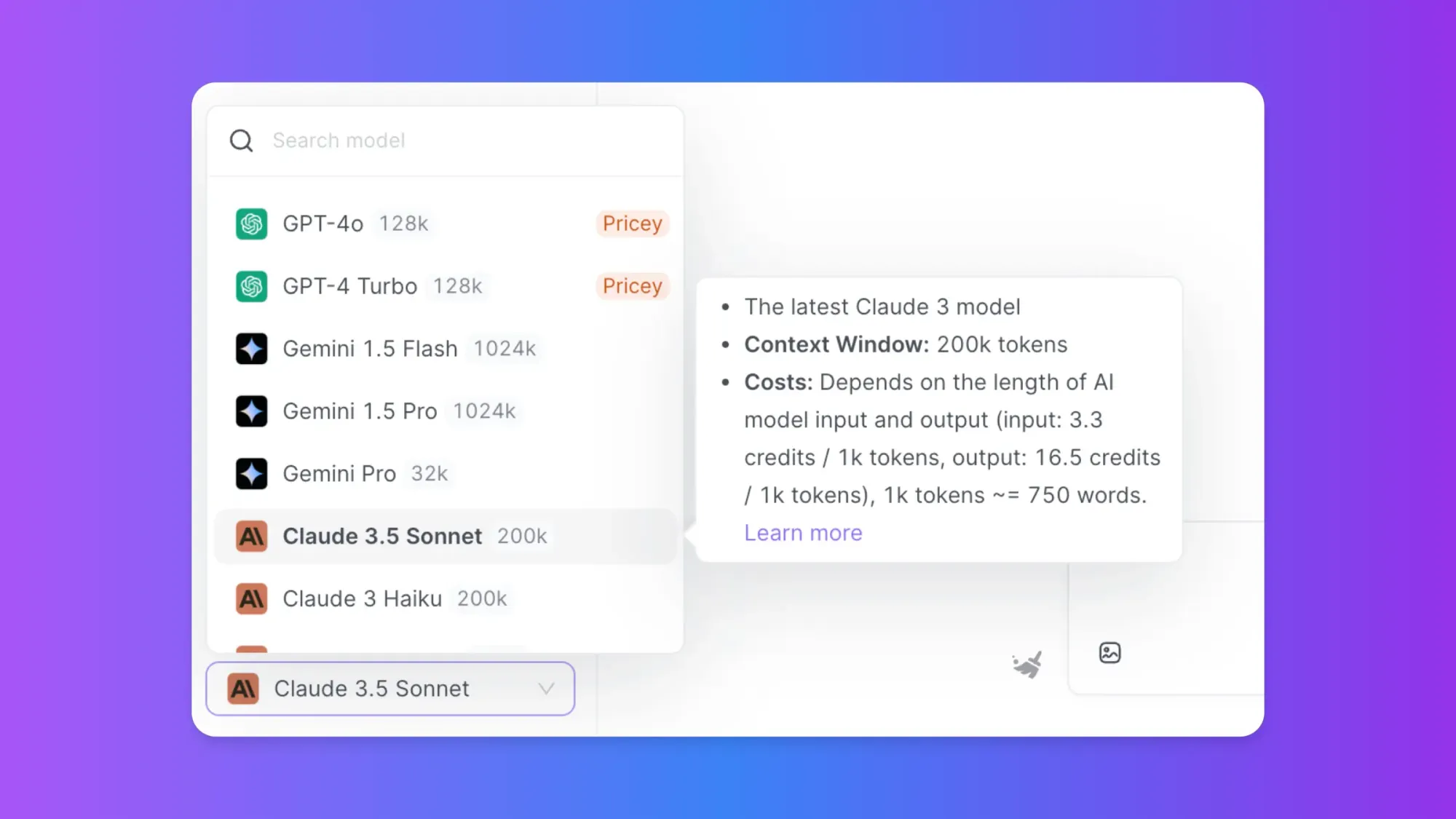
Installing Ollama
The first step in using Ollama is to install it on your system. Ollama supports macOS, Linux, and Windows (preview).
Downloading and Installing Ollama
To install Ollama:
- Visit the official Ollama website (https://ollama.ai/).
- Click on the "Download" button.
- Select the appropriate version for your operating system.
- Once downloaded, run the installer and follow the on-screen instructions.
For Linux users, you can use the following command to install Ollama:
curl -fsSL https://ollama.ai/install.sh | sh
After installation, you can verify that Ollama is working by opening a terminal and running:
ollama --version
Running Your First Model with Ollama
Once Ollama is installed, you can start using it to run LLMs locally.
Pulling and Running a Model with Ollama
To use a model, you first need to pull it from Ollama's model library. Let's start with the popular Llama 2 model:
ollama pull llama2
After the model is downloaded, you can run it using the following command:
ollama run llama2
This will start an interactive session where you can chat with the model. Try asking it a question:
>>> What is the capital of France?
The capital of France is Paris. Paris is the largest city in France and serves as the country's political, economic, and cultural center. It is known for its iconic landmarks such as the Eiffel Tower, the Louvre Museum, and Notre-Dame Cathedral. Paris has been the capital of France since the Middle Ages and has played a significant role in European history and culture.
>>> How can I learn to code?
Learning to code is an excellent skill to develop, and there are many ways to get started. Here are some steps you can take to begin your coding journey:
1. Choose a programming language: Start with a beginner-friendly language like Python, JavaScript, or Ruby.
2. Use online resources: Websites like Codecademy, freeCodeCamp, and Coursera offer free coding courses.
3. Practice regularly: Consistency is key when learning to code. Try to code a little bit every day.
4. Work on projects: Apply your skills by building small projects that interest you.
5. Join coding communities: Participate in forums, attend meetups, or join online coding groups for support and networking.
6. Read coding books: There are many great books for beginners that can supplement your learning.
7. Use coding apps: Mobile apps like SoloLearn or Grasshopper can help you practice on the go.
8. Take a bootcamp or course: Consider enrolling in a coding bootcamp or online course for structured learning.
9. Contribute to open-source projects: Once you have some skills, contributing to open-source projects can be a great way to gain experience.
10. Be patient and persistent: Learning to code takes time, so don't get discouraged if you face challenges.
Remember, everyone learns at their own pace, so find a method that works best for you and stick with it. Good luck on your coding journey!
Integrating Ollama with Python
Ollama can be easily integrated into Python applications, allowing you to leverage LLMs in your projects.
Setting Up Ollama with Python
First, install the Ollama Python library:
pip install ollama
Now, you can use Ollama in your Python scripts. Here's a simple example:
import ollama
# Generate a response
response = ollama.generate(model='llama2', prompt='What is the meaning of life?')
print(response['response'])
Creating a Simple Chatbot with Ollama
Let's create a more interactive chatbot using Ollama and Python:
import ollama
def chat_with_ollama():
print("Welcome to the Ollama Chatbot! Type 'exit' to end the conversation.")
while True:
user_input = input("You: ")
if user_input.lower() == 'exit':
print("Goodbye!")
break
response = ollama.generate(model='llama2', prompt=user_input)
print("Ollama:", response['response'])
if __name__ == "__main__":
chat_with_ollama()
This script creates a simple interactive chatbot that uses the Llama 2 model to generate responses.
Advanced Ollama Usage
Ollama offers more advanced features for power users and developers.
Using Different Models with Ollama
Ollama supports a variety of models. You can list available models and switch between them:
ollama list
ollama run mistral
ollama run vicuna
Fine-tuning Models with Ollama
Ollama allows you to fine-tune models for specific tasks. Here's a basic example of how to create a custom model:
- Create a Modelfile:
FROM llama2
# Set a custom system message
SYSTEM You are a helpful assistant specialized in programming.
# Add some training data
PROMPT What is Python?
RESPONSE Python is a high-level, interpreted programming language known for its simplicity and readability. It was created by Guido van Rossum and first released in 1991. Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming. It has a large and comprehensive standard library, making it suitable for a wide range of applications, from web development to data analysis and artificial intelligence.
# Set some parameters
PARAMETER temperature 0.7
PARAMETER top_k 40
PARAMETER top_p 0.95
- Create the model:
ollama create programming-assistant -f Modelfile
- Run the custom model:
ollama run programming-assistant
Using Ollama with REST API
Ollama provides a REST API that you can use to integrate it with other applications. Here's an example using Python's requests library:
import requests
import json
def generate_response(prompt):
url = "http://localhost:11434/api/generate"
data = {
"model": "llama2",
"prompt": prompt
}
response = requests.post(url, json=data)
return json.loads(response.text)['response']
# Example usage
prompt = "Explain quantum computing in simple terms."
response = generate_response(prompt)
print(response)
Building Applications with Ollama
Ollama's versatility allows you to build various applications. Let's explore a few examples.
Creating a Question-Answering System with Ollama
Here's a simple question-answering system using Ollama:
import ollama
def answer_question(question):
context = """
The solar system consists of the Sun and everything that orbits around it, including planets, moons, asteroids, comets, and meteoroids. There are eight planets in our solar system: Mercury, Venus, Earth, Mars, Jupiter, Saturn, Uranus, and Neptune. Pluto was once considered the ninth planet but was reclassified as a dwarf planet in 2006.
"""
prompt = f"Context: {context}\n\nQuestion: {question}\n\nAnswer:"
response = ollama.generate(model='llama2', prompt=prompt)
return response['response']
# Example usage
question = "How many planets are in our solar system?"
answer = answer_question(question)
print(f"Q: {question}")
print(f"A: {answer}")
Building a Text Summarization Tool with Ollama
Let's create a tool that summarizes long texts:
import ollama
def summarize_text(text):
prompt = f"Please summarize the following text in a concise manner:\n\n{text}\n\nSummary:"
response = ollama.generate(model='llama2', prompt=prompt)
return response['response']
# Example usage
long_text = """
Artificial intelligence (AI) is intelligence demonstrated by machines, as opposed to natural intelligence displayed by animals including humans. AI research has been defined as the field of study of intelligent agents, which refers to any system that perceives its environment and takes actions that maximize its chance of achieving its goals. The term "artificial intelligence" had previously been used to describe machines that mimic and display "human" cognitive skills that are associated with the human mind, such as "learning" and "problem-solving". This definition has since been rejected by major AI researchers who now describe AI in terms of rationality and acting rationally, which does not limit how intelligence can be articulated.
"""
summary = summarize_text(long_text)
print("Summary:", summary)
Optimizing Ollama Performance
To get the best performance out of Ollama, consider the following tips:
Hardware Considerations for Ollama
Ollama can run on CPUs, but it performs much better with GPU acceleration. If you're using Ollama for serious work, consider using a machine with a dedicated GPU.
Optimizing Model Selection in Ollama
Choose the right model for your task. Smaller models like Mistral or Phi-2 are faster but may be less capable. Larger models like Llama 2 70B are more powerful but require more resources.
Caching and Preloading Models with Ollama
Ollama automatically caches models, but you can preload models to reduce startup time:
ollama run llama2 < /dev/null
This command loads the model into memory without starting an interactive session.
Troubleshooting Common Ollama Issues
When using Ollama, you might encounter some issues. Here are solutions to common problems:
Resolving Model Download Issues in Ollama
If you're having trouble downloading models, try the following:
- Check your internet connection.
- Ensure you have enough disk space.
- Try using a VPN if your network is blocking the download.
Handling Out-of-Memory Errors with Ollama
If you encounter out-of-memory errors:
- Try using a smaller model.
- Increase your system's swap space.
- Upgrade your hardware, particularly RAM.
Addressing Slow Response Times in Ollama
If responses are slow:
- Use GPU acceleration if available.
- Reduce the
max_tokens
parameter for faster responses. - Consider using a smaller, faster model for less complex tasks.
Conclusion
Ollama is a powerful tool that brings the capabilities of large language models to your local machine. By following this guide, you should now be able to install Ollama, run models, integrate it with Python, and build applications using its capabilities. Remember to experiment with different models and settings to find the best configuration for your specific use case. As Ollama continues to evolve, keep an eye on the official documentation for new features and improvements. Happy coding with Ollama!
Then, You cannot miss out Anakin AI!
Anakin AI is an all-in-one platform for all your workflow automation, create powerful AI App with an easy-to-use No Code App Builder, with Llama 3, Claude Sonnet 3.5, GPT-4, Uncensored LLMs, Stable Diffusion...
Build Your Dream AI App within minutes, not weeks with Anakin AI!
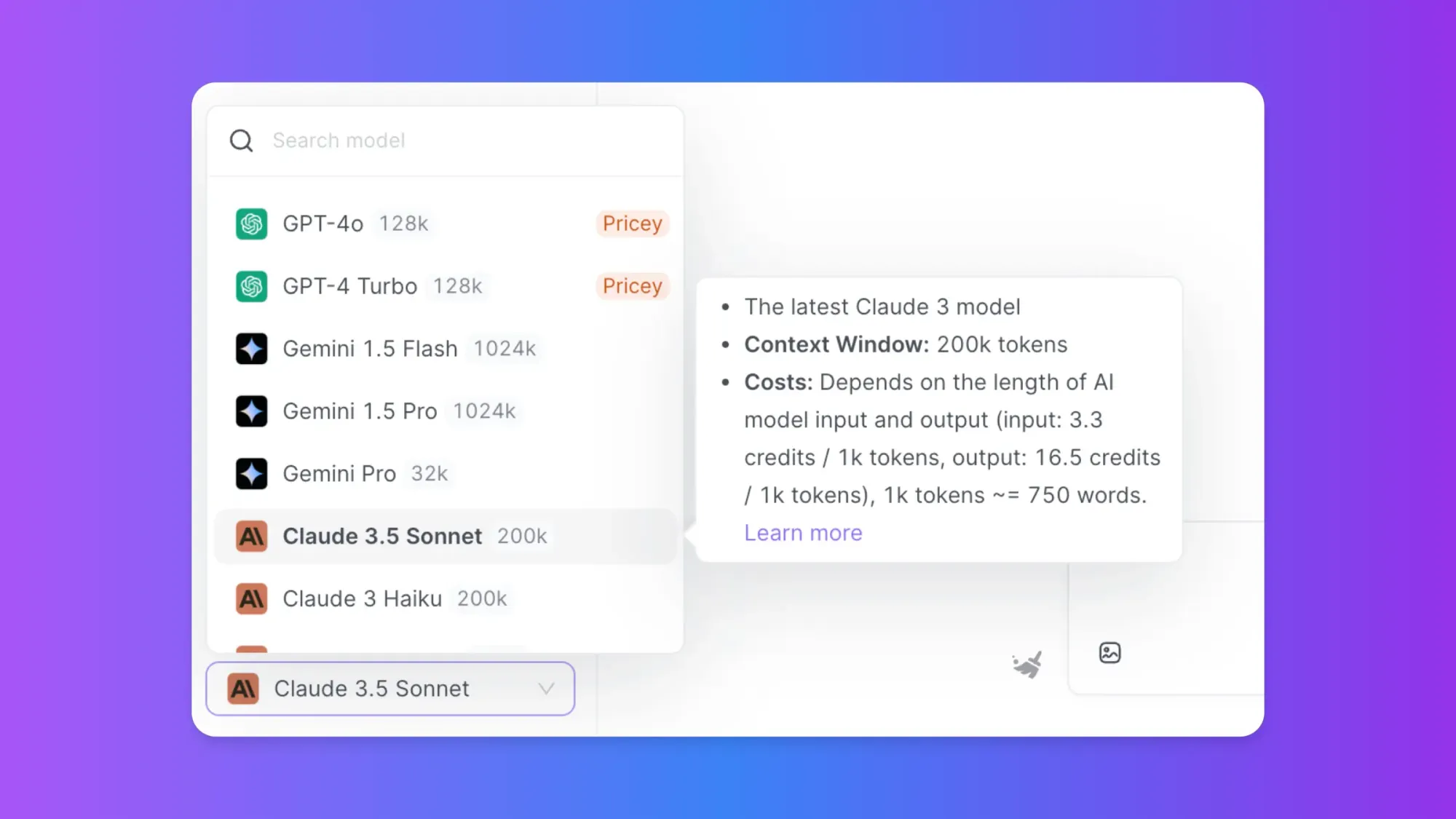