Web scraping has long been an essential tool for data collection and analysis. With the advent of Large Language Models (LLMs), we can now create more intelligent and adaptable web scrapers. This article will guide you through the process of building an LLM-powered web scraper, combining traditional scraping techniques with the power of language models.
Searching for an AI Platform that gives you access to any AI Model with an All-in-One price tag?
Then, You cannot miss out Anakin AI!
Anakin AI is an all-in-one platform for all your workflow automation, create powerful AI App with an easy-to-use No Code App Builder, with Llama 3, Claude, GPT-4, Uncensored LLMs, Stable Diffusion...
Build Your Dream AI App within minutes, not weeks with Anakin AI!
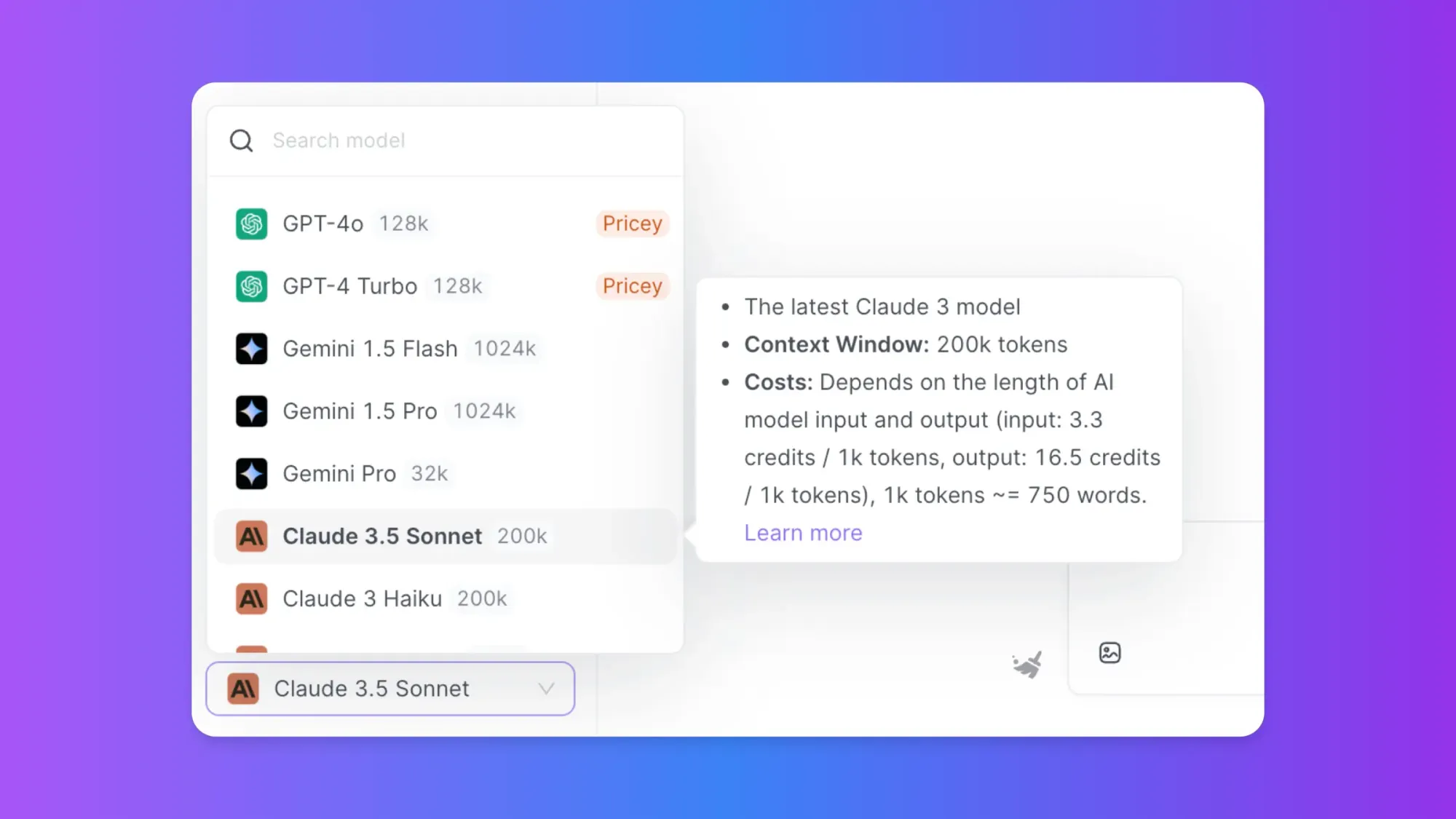
Introduction to LLM Web Scrapers
LLM web scrapers leverage the capabilities of large language models to understand and extract information from web pages more effectively than traditional scrapers. They can adapt to changes in website structures, understand context, and even extract implicit information that might be challenging for rule-based scrapers.
Prerequisites
Before we begin, ensure you have the following installed:
- Python 3.7+
- pip (Python package manager)
Step 1: Setting Up the Environment
First, let's create a virtual environment and install the necessary libraries:
python -m venv llm_scraper_env
source llm_scraper_env/bin/activate # On Windows, use: llm_scraper_env\Scripts\activate
pip install requests beautifulsoup4 langchain openai
Step 2: Basic Web Scraping Setup
Let's start with a basic web scraping setup using requests and BeautifulSoup:
import requests
from bs4 import BeautifulSoup
def fetch_webpage(url):
response = requests.get(url)
if response.status_code == 200:
return response.text
else:
raise Exception(f"Failed to fetch the webpage. Status code: {response.status_code}")
def parse_html(html_content):
return BeautifulSoup(html_content, 'html.parser')
# Example usage
url = "https://example.com"
html_content = fetch_webpage(url)
soup = parse_html(html_content)
# Basic extraction
title = soup.title.string if soup.title else "No title found"
paragraphs = [p.text for p in soup.find_all('p')]
print(f"Title: {title}")
print(f"Number of paragraphs: {len(paragraphs)}")
This basic setup fetches a webpage and parses its HTML content. However, it lacks the intelligence to adapt to different webpage structures or extract complex information.
Step 3: Integrating an LLM
Now, let's integrate an LLM to enhance our scraper's capabilities. We'll use OpenAI's GPT model through the LangChain library:
import os
from langchain.llms import OpenAI
from langchain.prompts import PromptTemplate
from langchain.chains import LLMChain
# Set your OpenAI API key
os.environ["OPENAI_API_KEY"] = "your-api-key-here"
def create_llm_chain():
llm = OpenAI(temperature=0.7)
prompt_template = PromptTemplate(
input_variables=["html_content", "extraction_task"],
template="Given the following HTML content:\n{html_content}\n\nExtract the following information: {extraction_task}"
)
return LLMChain(llm=llm, prompt=prompt_template)
llm_chain = create_llm_chain()
def extract_with_llm(html_content, extraction_task):
return llm_chain.run(html_content=html_content, extraction_task=extraction_task)
# Example usage
extraction_task = "1. The main headline\n2. A list of all product names\n3. The price of each product"
result = extract_with_llm(html_content, extraction_task)
print(result)
This setup uses an LLM to interpret the HTML content and extract specific information based on the given task.
Step 4: Enhancing Extraction with HTML Cleaning
To improve the LLM's performance, let's clean the HTML before passing it to the model:
import re
def clean_html(html_content):
# Remove script and style elements
soup = BeautifulSoup(html_content, 'html.parser')
for script in soup(["script", "style"]):
script.decompose()
# Get text content
text = soup.get_text()
# Break into lines and remove leading and trailing space on each
lines = (line.strip() for line in text.splitlines())
# Break multi-headlines into a line each
chunks = (phrase.strip() for line in lines for phrase in line.split(" "))
# Drop blank lines
text = '\n'.join(chunk for chunk in chunks if chunk)
return text
# Modify the extract_with_llm function
def extract_with_llm(html_content, extraction_task):
cleaned_content = clean_html(html_content)
return llm_chain.run(html_content=cleaned_content, extraction_task=extraction_task)
This cleaning process removes unnecessary HTML elements and formatting, making it easier for the LLM to focus on the relevant content.
Step 5: Implementing Adaptive Scraping
One of the key advantages of using an LLM is its ability to adapt to different webpage structures. Let's implement a function that can scrape multiple pages with varying layouts:
def adaptive_scrape(urls, extraction_task):
results = []
for url in urls:
try:
html_content = fetch_webpage(url)
extracted_info = extract_with_llm(html_content, extraction_task)
results.append({"url": url, "extracted_info": extracted_info})
except Exception as e:
print(f"Error scraping {url}: {str(e)}")
return results
# Example usage
urls = [
"https://example.com/product1",
"https://example.com/product2",
"https://anothersite.com/item"
]
extraction_task = "Extract the product name, price, and a brief description"
scraping_results = adaptive_scrape(urls, extraction_task)
for result in scraping_results:
print(f"URL: {result['url']}")
print(f"Extracted Info:\n{result['extracted_info']}\n")
This adaptive scraping function can handle multiple URLs with different structures, using the same extraction task for all of them.
Step 6: Implementing Structured Data Extraction
While free-form text extraction is powerful, sometimes we need structured data. Let's modify our LLM chain to output JSON:
import json
def create_structured_llm_chain():
llm = OpenAI(temperature=0.7)
prompt_template = PromptTemplate(
input_variables=["html_content", "extraction_task"],
template="Given the following HTML content:\n{html_content}\n\nExtract the following information: {extraction_task}\n\nOutput the result as a valid JSON object."
)
return LLMChain(llm=llm, prompt=prompt_template)
structured_llm_chain = create_structured_llm_chain()
def extract_structured_data(html_content, extraction_task):
cleaned_content = clean_html(html_content)
result = structured_llm_chain.run(html_content=cleaned_content, extraction_task=extraction_task)
try:
return json.loads(result)
except json.JSONDecodeError:
print("Failed to parse LLM output as JSON. Raw output:")
print(result)
return None
# Example usage
extraction_task = "product_name, price, description"
structured_data = extract_structured_data(html_content, extraction_task)
if structured_data:
print(json.dumps(structured_data, indent=2))
This modification ensures that the LLM outputs structured JSON data, making it easier to process and store the extracted information.
Step 7: Handling Pagination and Dynamic Content
Many websites use pagination or load content dynamically. Let's enhance our scraper to handle these scenarios:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
def fetch_dynamic_webpage(url, wait_for_element=None, scroll=False):
options = webdriver.ChromeOptions()
options.add_argument('--headless')
driver = webdriver.Chrome(options=options)
try:
driver.get(url)
if wait_for_element:
WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.CSS_SELECTOR, wait_for_element))
)
if scroll:
# Scroll to the bottom of the page
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
# Wait for any lazy-loaded content
import time
time.sleep(2)
return driver.page_source
finally:
driver.quit()
def scrape_paginated_content(base_url, num_pages, extraction_task):
all_data = []
for page in range(1, num_pages + 1):
url = f"{base_url}?page={page}"
html_content = fetch_dynamic_webpage(url, wait_for_element='.product-list', scroll=True)
page_data = extract_structured_data(html_content, extraction_task)
if page_data:
all_data.extend(page_data)
return all_data
# Example usage
base_url = "https://example.com/products"
num_pages = 3
extraction_task = "Extract an array of products, each with: name, price, rating"
paginated_data = scrape_paginated_content(base_url, num_pages, extraction_task)
print(json.dumps(paginated_data, indent=2))
This enhancement uses Selenium to handle dynamic content and pagination, allowing the scraper to extract data from more complex websites.
Step 8: Implementing Rate Limiting and Error Handling
To be a responsible scraper and handle potential issues, let's add rate limiting and robust error handling:
import time
from ratelimit import limits, sleep_and_retry
@sleep_and_retry
@limits(calls=1, period=5) # Limit to 1 call every 5 seconds
def rate_limited_fetch(url):
return fetch_webpage(url)
def scrape_with_retries(url, extraction_task, max_retries=3):
for attempt in range(max_retries):
try:
html_content = rate_limited_fetch(url)
return extract_structured_data(html_content, extraction_task)
except Exception as e:
print(f"Error on attempt {attempt + 1}: {str(e)}")
if attempt == max_retries - 1:
print(f"Failed to scrape {url} after {max_retries} attempts")
return None
time.sleep(5) # Wait before retrying
# Example usage
url = "https://example.com/product"
extraction_task = "product_name, price, availability"
result = scrape_with_retries(url, extraction_task)
if result:
print(json.dumps(result, indent=2))
This implementation adds rate limiting to prevent overwhelming the target website and includes retry logic to handle temporary failures.
Conclusion
Building an LLM-powered web scraper combines the flexibility of traditional web scraping techniques with the intelligence of large language models. This approach allows for more adaptive and robust data extraction, capable of handling various website structures and content types.
By following the steps outlined in this guide, you can create a powerful web scraping tool that leverages the capabilities of LLMs. Remember to always scrape responsibly, respecting website terms of service and implementing appropriate rate limiting.
As you continue to develop your LLM web scraper, consider exploring advanced features such as:
- Implementing a user interface for easy task definition
- Storing scraped data in a database for further analysis
- Creating a pipeline for regular, automated scraping tasks
- Fine-tuning the LLM on specific domains for improved accuracy
With these enhancements, your LLM web scraper will be a valuable tool for data collection and analysis in various fields and applications.
Searching for an AI Platform that gives you access to any AI Model with an All-in-One price tag?
Then, You cannot miss out Anakin AI!
Anakin AI is an all-in-one platform for all your workflow automation, create powerful AI App with an easy-to-use No Code App Builder, with Llama 3, Claude, GPT-4, Uncensored LLMs, Stable Diffusion...
Build Your Dream AI App within minutes, not weeks with Anakin AI!
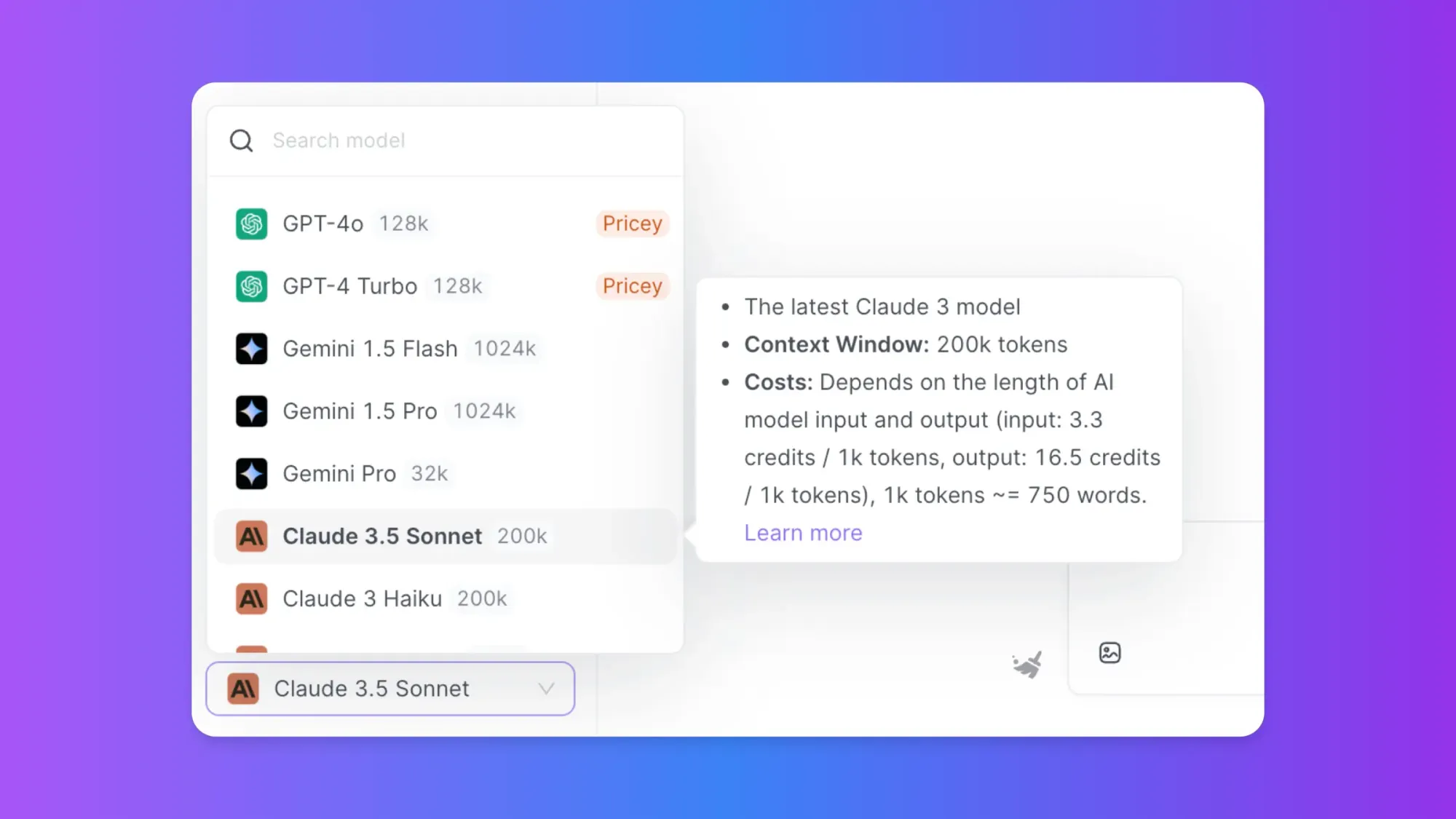